~ by RAKA on 04-August-2023
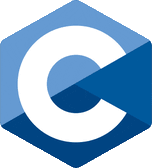
~ by RAKA on 04-August-2023
As we head into the era of Data Science, programmers are going to be revered like Modern-day Gods. No, seriously—our job is to organize the chaos of endless data. But just like gods, we need the right tools to manage this data. Enter: Collections in C.
So, sit back, stay calm, and let me show you how to take control of your data like a programming deity.
1. What is a Collection?
Imagine you’re building a Color Picker program, and you need to assign 100 different colors. Or, let’s say you need to manage data for 100 employees, including their age, designation, and name. Without collections, you'd need a hundred separate variables. Sounds like a headache, right?
That’s where collections in C come in—saving you from a world of pain. A collection groups together logically related data and stores it in a way that’s easier to manage. Think of it like keeping your kitchen utensils in one drawer instead of scattering them all over the house.
2. Types of Collections in C
Basically, you’ve got two types of collections in C:
1. Collections with the same data type: Enter the Array.
2. Collections with different data types: Meet the Structure.
You either stick with one flavor or mix them up. Let’s dive into both.
3. Arrays: The Loyal Soldiers
An Array is a collection of elements that are all of the same data type. Like a neat line of soldiers, they’re ordered and reliable.
Syntax:
data_type array_name[array_size] = { elements ... };
• Array Name: Think of it as a variable name, but for a group of elements.
• Array Size: The number of elements you can store, just like how many seats are in a bus.
3.1. First Array Example
int arr[10]; // Stores 10 integers, indexed from 0 to 9
Your array will look something like this:
arr[0], arr[1], arr[2], ..., arr[9]
Remember, array indices start from 0. So, if you declare an array of size 10, the valid indices are from arr[0] to arr[9]. Yeah, it’s not exactly intuitive, but that’s life.
3.2. Array Declaration
int num[5] = {10, 20, 30, 40, 50}; // Declaring with size and values
float rates[] = {2.5, 3.5, 4.5}; // Without size, but values are required
char letters[5]; // Size defined, values will come later
Pro-tip: If you don’t fill up all the spots, C will kindly assign 0 to the remaining spaces. Talk about a helpful friend!
3.3. Accessing Array Elements
Once you’ve set up your array, you can manipulate it like a boss:
int total = num[0] + num[1]; // Adds the first two elements
printf("%d", num[2]); // Prints the third element
Want to find out how big your array is? Use the sizeof() function:
int size = sizeof(num) / sizeof(int); // Gets the number of elements in the array
Structures: The Mix-and-Match Masterpiece
4. Collections in C: Wrap-Up
Collections are like your loyal team members—they organize your data and keep it manageable. Arrays will be your go-to when you're dealing with the same data type, while structures will come in handy when you need to mix and match.
So go ahead, embrace collections and bring order to the chaos in your programs. After all, even gods need a system to run their worlds smoothly.
Happy coding, future programming deity! 😎