~ by RAKA on 03-August-2023
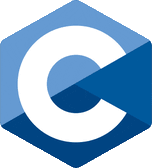
~ by RAKA on 03-August-2023
So, here we are, in the dark world of programming, staring at a black screen while dreaming of creating colorful, functional software that dazzles the masses. You know what? You’re not just a user; you’re a programmer—the mastermind behind the curtain. Welcome to the power of variables, your new best friends in C programming.
1. What is a Variable?
You remember those pesky algebra classes where “X” was the mystery we all pretended to care about? Well, in programming, a variable is your "X", but with a way cooler job. Think of it as a named storage location in your computer's memory where you can stash some data, only to use and manipulate later.
Not just any random stash though; in C, we follow a specific formula to declare a variable:
That's it! Simple, yet so powerful.
2. The Real Deal: How Variables Work
So, what actually happens when you declare a variable? Let's break it down for you, my future coding warrior:
Boom, done! You just bossed your memory around like it’s your kingdom. But what if you don’t give it a value? Well, in that case, the variable contains some garbage value (nope, not your junk email folder, but actual unwanted data).
Now your "some" has a purpose! Go ahead, feel proud.
3. Popular Variable Naming Styles: The Cool Kid’s Club
Now that you're controlling memory like a pro, you might as well do it in style. There are two popular ways to name your variables:
• camelCase: firstName, totalScore, numberOfStudents
• snake_case: first_name, total_score, number_of_students
or you may creat your own style. Do whatever fits your style, just make sure you don’t mix them up like a confused snake-camel hybrid.
4. Do’s and Don’ts of Variable Naming
Let’s get serious for a second. You’re a professional programmer, and you need to look the part. So here are a few rules:
• Use descriptive names: Please, for the love of all things code, don’t name your variables like they’re secret agents. Use total_students instead of just total. Future-you will thank you.
• Start with a letter: Naming your variable 1_student is a big no-no. Instead, try student_1.
• Avoid Reserved Keywords: You might think naming a variable int or float is clever, but trust me, it’s not. Those are reserved-Keywords, you cant use them as variable.
5. Scope of a Variable: Global vs. Local
Here’s where things get a little technical but stay with me—it's important. A variable's scope defines where in your code the variable can be accessed.
• Global: Available everywhere in your program (but careful, too much freedom can be dangerous!).
• Local: Only available within the specific block or function where it’s declared.
And just like that, you're in control. You decide where and when your variables can be accessed. Feels good, doesn't it?
6. Variable Casting: Shape-Shifting in C
Alright, now that you're a memory magician, let’s take things up a notch with casting. This is the magic that allows you to change the data type of a variable.
Example:
Pretty neat, huh? With casting, your variables can transform at will, like a digital shape-shifter.
7. The Golden Rule: Initialize Your Variables
Here’s a quick piece of wisdom: always initialize your variables. Assign them something, even if it’s just 0. Why? Because uninitialized variables are ticking time bombs filled with garbage. Trust me, no one likes dealing with garbage—unless you're an actual garbage collector (but that’s a whole other field of study).
8. Example Code: Simple Interest Calculator
Let’s take everything you’ve learned and put it to use in a quick example. You’ll be calculating simple interest in no time.
And just like that, you’ve created a simple interest calculator. Go on, show off your new skills.
9. Conclusion
Variables are more than just containers for data; they’re the building blocks of logic and functionality in C programming. Once you understand how to control, manipulate, and cast them, you’re one step closer to becoming a master coder.
So, keep coding, keep learning, and most importantly—love the black screen. It’s where the magic happens!