~ by RAKA on 04-August-2023
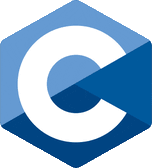
~ by RAKA on 04-August-2023
As we head into the era of Data Science, programmers are going to be revered like Modern-day Gods. No, seriously—our job is to organize the chaos of endless data. But just like gods, we need the right tools to manage this data. Enter: Collections in C.
So, sit back, stay calm, and let me show you how to take control of your data like a programming deity.
1. What is a Collection?
Imagine you’re building a Color Picker program, and you need to assign 100 different colors. Or, let’s say you need to manage data for 100 employees, including their age, designation, and name. Without collections, you'd need a hundred separate variables. Sounds like a headache, right?
That’s where collections in C come in—saving you from a world of pain. A collection groups together logically related data and stores it in a way that’s easier to manage. Think of it like keeping your kitchen utensils in one drawer instead of scattering them all over the house.
2. Types of Collections in C
Basically, you’ve got two types of collections in C:
1. Collections with the same data type: Enter the Array.
2. Collections with different data types: Meet the Structure.
You either stick with one flavor or mix them up. Let’s dive into both.
3. Structures: The Mix-and-Match Masterpiece
Now, arrays are great for single data types, but what if you need to store multiple data types together? Meet Structures, the swiss army knife of C collections.
3.1. Syntax:
struct structure_name {
data_type member1;
data_type member2;
...
} instance1, instance2;
Structures are like little baskets where you can mix and match different types of variables, all under one roof.
3.2. Example: The Mighty Product Structure
struct Product {
char quality;
int quantity;
};
struct Product p1; // Declaring a variable of type Product
p1.quantity = 20; // Accessing and assigning values
Here’s the fun part: you can create Nested Structures too, because why not? Let’s say you have a product with a manufacturing date and expiration date—both of which have month and year.
Here’s how you can handle that:
struct Date {
int month;
int year;
};
struct Product {
int sl_no;
struct Date mfg_date;
struct Date exp_date;
};
struct Product p1;
p1.mfg_date.year = 2023;
p1.exp_date.year = 2025;
Boom! You’ve just nested a structure inside another structure like a pro.
4. Time for Some Code: Build a Simple Database
Want to see all this in action? Let’s build a Product Database using structures. You’ll define a product with serial number, manufacturing date, expiration date, and quality. Then, you’ll input new products and display the full product list.
Here’s the skeleton code:
#include <stdio.h>
struct Date {
int month;
int year;
};
struct Product {
int sl_no;
struct Date mfg_date;
struct Date exp_date;
char quality;
};
void main() {
struct Product p1;
// Input data for p1
p1.sl_no = 1;
p1.mfg_date.year = 2023;
p1.exp_date.year = 2025;
p1.quality = 'A';
// Output product details
printf("Product Serial No: %d\n", p1.sl_no);
printf("Manufacture Year: %d\n", p1.mfg_date.year);
printf("Expiry Year: %d\n", p1.exp_date.year);
printf("Quality: %c\n", p1.quality);
}
5. Collections in C: Wrap-Up
Collections are like your loyal team members—they organize your data and keep it manageable. Arrays will be your go-to when you're dealing with the same data type, while structures will come in handy when you need to mix and match.
So go ahead, embrace collections and bring order to the chaos in your programs. After all, even gods need a system to run their worlds smoothly.
Happy coding, future programming deity! 😎