~ by RAKA on 02-August-2023
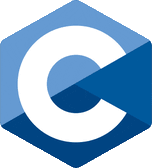
~ by RAKA on 02-August-2023
When programming in C, one of the foundational concepts is understanding data types. Data types in C determine what kind of data a variable can store. Whether it's numbers, characters, or floating-point numbers, knowing how data is stored and processed is essential for writing efficient programs.
1. What is Data-Type?
In the simplest terms, a data-type refers to any piece of information that can be stored, processed, or transmitted. Data can be classified broadly into two categories:
• Numbers: These can be integers (like 0, 1, 1000) or floating-point numbers (like 1.1, -15.005).
• Alphabets: This can be a single Character (like 'A', 'b', '@') or a string (like “Hello World@123”).
Understanding these different types of data and how they are handled in C is crucial for efficient coding.
2. Basic Data Types in C
In C, we have several basic data types:
2.1. Numeric Types:
• Integer (int): Used for whole numbers like 5, -100, etc.
• Floating Point(float): Used for numbers with decimals, such as 2.6, -3.14.
• Double (double): For large floating-point numbers requiring higher precision.
2.2. Alphabetic Types:
• Character (int): Represents single characters like 'A' or '#'.
• String (int): Not a data type by itself but essentially a collection of characters (like char[]).
Each data type has its own memory size and range, allowing the programmer to choose the best type for the given application.
3. Memory Size and Range of Data Types
Understanding how much memory each data type occupies is critical for writing efficient code and managing memory properly in large-scale applications.
• int: Size: 4Bytes, Range: -2,147,483,648 to 2,147,483,647
• float: Size: 4Bytes, Range: ±(3.4 x 10-38) to ±(3.4 x 1038)
• double: Size: 8Bytes, Range: ±(2.22507 x 10-308) to ±(1.79769 x 10308)
• char: Size: 1Bytes, Stores a character's ASCII value (0–255)
As a C programmer, you need to be conscious of how much memory your program uses, especially when dealing with larger datasets.
4. Understanding sizeof() in C
The sizeof() operator is a valuable tool that helps you find out the size of a data type or variable in terms of the memory it occupies. The output is an integer that represents the memory size in bytes.
4.1. Why Is This Important?
Memory is limited, especially in systems programming, embedded systems, or mobile apps. Being aware of the memory size of each data type helps ensure you’re using your resources efficiently.
4.2. Code Example: Using sizeof() to Find Data Type Sizes
Let’s write a simple C program that demonstrates how to use the sizeof() operator to check the memory size of different data types.
#include <stdio.h>
void main() {
printf("Size of int: %d bytes\n", sizeof(int));
printf("Size of float: %d bytes\n", sizeof(float));
printf("Size of double: %d bytes\n", sizeof(double));
printf("Size of char: %d byte\n", sizeof(char));
}
Explanation:
• sizeof(int) returns the size of the int data type (usually 4 bytes).
• You can replace int with float, double, or char to find the respective sizes.
By running this code, you’ll get a quick overview of the memory sizes in bytes for each of the basic C data types.
5. Conclusion
Understanding data types in C is the foundation of programming. Each data type serves a specific purpose and knowing when and where to use them will make your programs more efficient and effective. From integers to floating points and characters, managing data types properly ensures that your program runs smoothly without wasting valuable memory.
So, keep experimenting with different data types, and remember, coding in C is all about knowing the details that help you write better, faster programs.